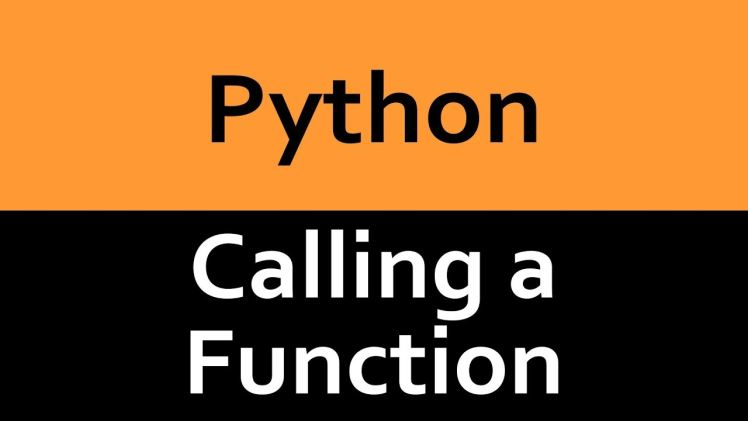
Introduction
Python, being a versatile programming language, offers many ways to efficiently perform various computing tasks. One of the essential elements of Python programming is the use of functions. Functions allow you to break down complex problems into smaller, manageable parts and reuse code. In this article, you will learn how to call a function in Python step by step.
Just remember, education is the doorway to happiness. To receive a good education, you will need good teachers. Also, you will need to be up on the latest tech.
What is a Function?
A function is a block of organized, reusable code that performs a specific task. Functions help improve modularity and facilitate code reusability. In Python, functions can be classified into built-in functions (predefined in Python) and user-defined functions (created by the programmer). Examples of built-in functions include print(), len(), and type().
Steps to Call a Function in Python:
- Define the Function
The first step to call a function in Python is defining the function using the ‘def’ keyword followed by the function name and parentheses containing any parameters (if required). The following line is an example of defining a simple function called greet:
“`python
def greet():
“`
- Add Function Body
After defining the function’s name and any necessary parameters, you need to write the function’s body. The body consists of indented statements that perform specific operations.
For example, here is our completed greet() function:
“`python
def greet():
print(“Hello, World!”)
“`
- Call the Function
To call a function in Python, you need to write the name of the function followed by parentheses containing any necessary arguments (if present).
In our example, we can call our ‘greet()’ function like this:
“`python
greet()
“`
When executed, this will print “Hello, World!” on screen.
- Call Functions With Parameters
If your function has parameters, you need to provide corresponding arguments when calling it.
For instance, let’s make a more personalized version of the greet function that accepts a ‘name’ parameter:
“`python
def greet(name):
print(“Hello, ” + name + “!”)
“`
To call this function with an argument, you need to provide the name when invoking it:
“`python
greet(“Alice”)
“`
When executed, this will print “Hello, Alice!” on screen.
Conclusion
Functions are an essential component of Python programming, enabling you to write more organized and reusuable code. In this article, you learned how to define and call functions in Python, including those with parameters. As you work with Python, functions will become an integral part of your coding practice, helping streamline your projects while increasing their efficiency and maintainability.